The Anagram Checker JavaScript Coding Challenge

- Published on
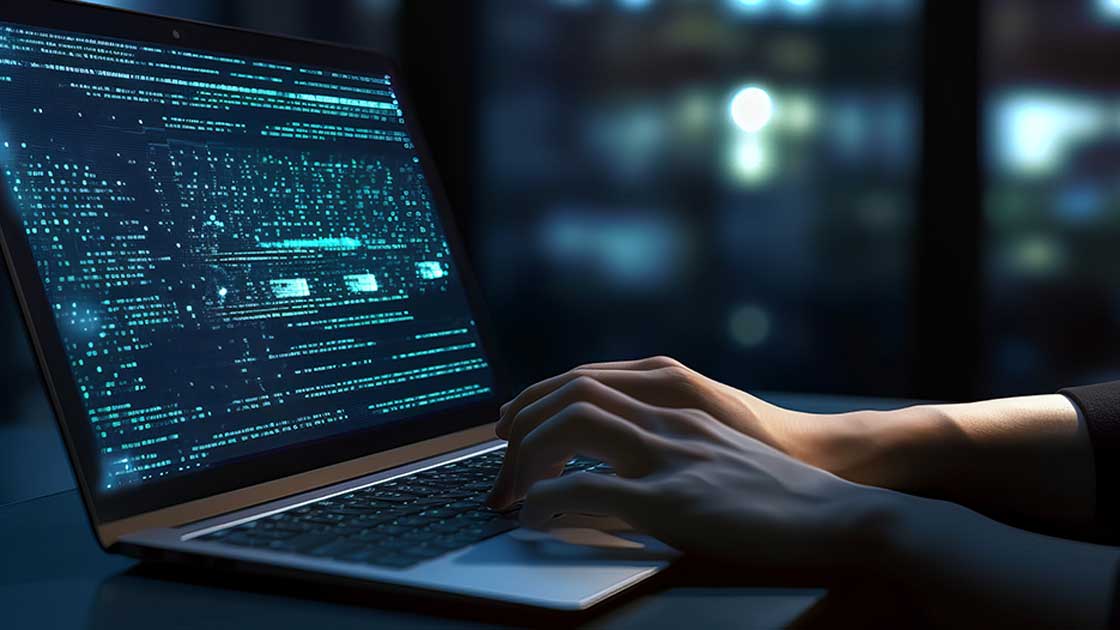
Are you up for a coding challenge that will put your JavaScript skills to the test? In this challenge, we will tackle the task of checking if two strings are anagrams. Anagrams are words or phrases formed by rearranging the letters of another word or phrase. It's a fun and challenging problem that requires attention to detail and algorithmic thinking. Let's dive in and see if you can build an anagram checker in JavaScript!
The Challenge
Write a JavaScript function that takes in two strings as parameters and determines if they are anagrams of each other. An anagram is a word or phrase that can be formed by rearranging the letters of another word or phrase. Your function should return true
if the strings are anagrams and false
otherwise.
For example:
- "listen" and "silent" are anagrams.
- "hello" and "world" are not anagrams.
The Solution
Let's solve the anagram checker challenge together. We'll start by writing a function called isAnagram
that takes in two strings and checks if they are anagrams.
function isAnagram(str1, str2) {
// Remove any non-alphabetic characters and convert to lowercase
const cleanStr1 = str1.replace(/[^A-Za-z]/g, '').toLowerCase()
const cleanStr2 = str2.replace(/[^A-Za-z]/g, '').toLowerCase()
// Sort the characters in the strings
const sortedStr1 = cleanStr1.split('').sort().join('')
const sortedStr2 = cleanStr2.split('').sort().join('')
// Compare the sorted strings
return sortedStr1 === sortedStr2
}
// Testing the function
console.log(isAnagram('listen', 'silent')) // true
console.log(isAnagram('hello', 'world')) // false
Explanation In the isAnagram
function, we first remove any non-alphabetic characters from the strings using regular expressions and convert them to lowercase. This step ensures that we compare only the letters in the strings.
Next, we split the cleaned strings into arrays of characters, sort the arrays, and join them back into strings. Sorting the characters allows us to easily compare if they are anagrams by checking if the sorted strings are equal.
Finally, we return true
if the sorted strings are equal (indicating an anagram) and false
otherwise.
Hi there! Want to support my work?
Congratulations on completing the anagram checker coding challenge! This challenge required you to employ string manipulation and algorithmic thinking to determine if two strings are anagrams. As you continue to solve coding challenges, you'll develop a stronger understanding of JavaScript and improve your problem-solving skills. Keep challenging yourself, exploring new coding concepts, and have fun on your coding journey!
Here is the complete solution on CodePen.