Efficient Techniques to Check if a Key Exists in JavaScript Objects (ES6+)

- Published on
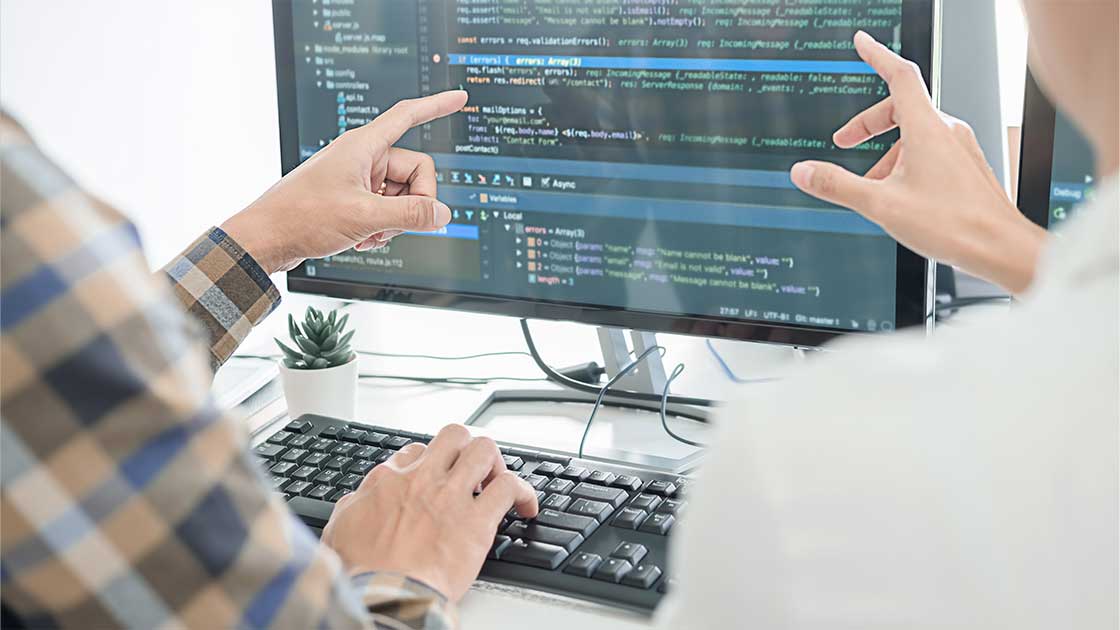
In modern JavaScript, specifically with the introduction of ES6 (ECMAScript 2015) and later versions, several methods are available to check if a key exists in an object. These methods provide concise and efficient ways to perform this common task. In this article, we will explore different techniques using ES6+ syntax to check if a key exists in JavaScript objects.
- Using the
in
operator: Thein
operator can be used to check if a key exists in an object. It returnstrue
if the key is present andfalse
otherwise. Here's an example:
const obj = { name: 'John', age: 25 }
console.log('name' in obj) // Output: true
console.log('address' in obj) // Output: false
- Using the
hasOwnProperty()
method: ThehasOwnProperty()
method is a built-in method available on objects. It returnstrue
if the object has a property with the specified key andfalse
otherwise. Here's an example:
const obj = { name: 'John', age: 25 }
console.log(obj.hasOwnProperty('name')) // Output: true
console.log(obj.hasOwnProperty('address')) // Output: false
- Using the
Reflect.has()
method: TheReflect.has()
method is part of theReflect
object, which provides methods for interceptable JavaScript operations. It can also be used to check if a key exists in an object. Here's an example:
const obj = { name: 'John', age: 25 }
console.log(Reflect.has(obj, 'name')) // Output: true
console.log(Reflect.has(obj, 'address')) // Output: false
- Using the optional chaining operator (
?.
): Introduced in ES2020, the optional chaining operator (?.
) allows you to access nested properties of an object without causing an error if any intermediate property isnull
orundefined
. It can be used to check if a key exists in a deeply nested object. Here's an example:
const obj = { person: { name: 'John', age: 25 } }
console.log(obj?.person?.name) // Output: 'John'
console.log(obj?.person?.address) // Output: undefined
By utilizing these methods, you can easily check if a key exists in a JavaScript object. Depending on your specific use case, you can choose the method that best fits your needs. Remember to consider browser compatibility when using newer syntax introduced in ES6 and later versions. With these techniques in your toolkit, you'll have no trouble verifying the presence of keys in JavaScript objects using modern syntax.
View the code on CodePen: https://codepen.io/utsukushiikilla/pen/oNQxZzO
Hi there! Want to support my work?