Mastering Fibonacci - A Guide to Solving Fibonacci Series Using Modern JavaScript (ES6+)

- Published on
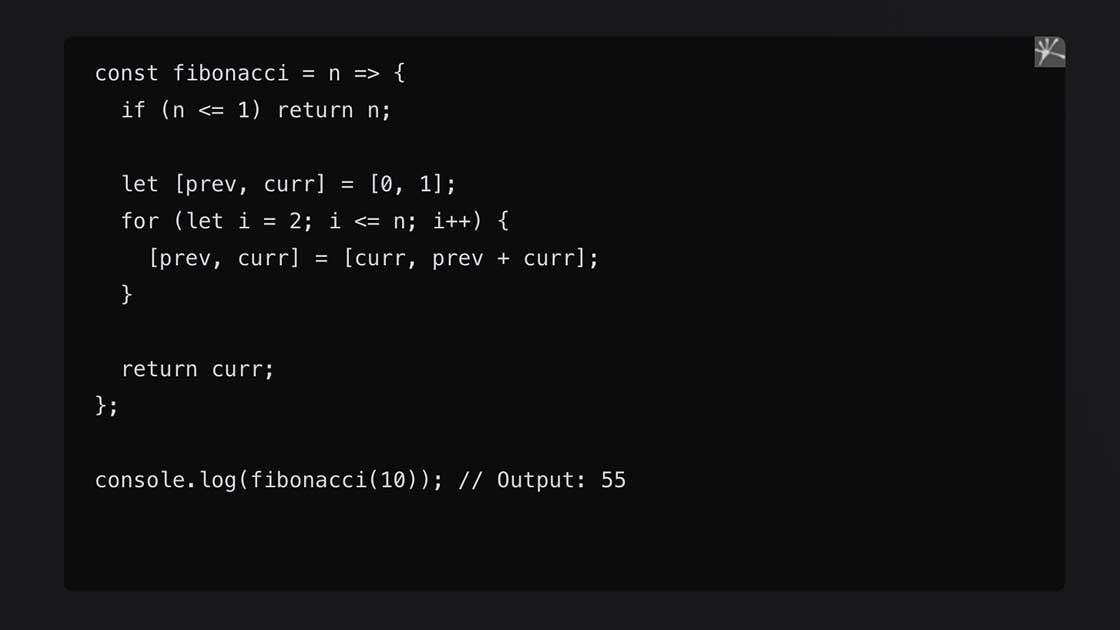
The Fibonacci sequence is a series of numbers in which each number is the sum of the two preceding ones, usually starting with 0 and 1. In this article, we'll explore how to solve the Fibonacci series using modern JavaScript (ES6 and later). We'll discuss three different approaches: iterative, recursive, and memoization, highlighting the pros and cons of each method.
Iterative Approach
The iterative approach is a straightforward method for generating the Fibonacci series using a loop. Here's a simple implementation using a for loop and ES6 features like arrow functions and destructuring assignment:
const fibonacci = (n) => {
if (n <= 1) return n
let [prev, curr] = [0, 1]
for (let i = 2; i <= n; i++) {
;[prev, curr] = [curr, prev + curr]
}
return curr
}
console.log(fibonacci(10)) // Output: 55
Pros:
- Easy to understand and implement
- Time complexity: O(n)
Cons:
- Not as elegant as other solutions
Recursive Approach
The recursive approach involves breaking down the problem into smaller subproblems, solving them, and combining the results. Here's the implementation using recursion, arrow functions, and the ternary operator:
const fibonacci = (n) => (n <= 1 ? n : fibonacci(n - 1) + fibonacci(n - 2))
console.log(fibonacci(10)) // Output: 55
Pros:
- Simple and elegant code
Cons:
- Inefficient: time complexity of O(2^n) due to repetitive calculations
Memoization
Memoization is an optimization technique that stores the results of expensive function calls and returns the cached results when the same inputs occur again. This approach significantly improves the recursive solution's performance. Here's the implementation using memoization, arrow functions, and destructuring assignment:
const fibonacci = (n, memo = {}) => {
if (n <= 1) return n
if (memo[n]) return memo[n]
memo[n] = fibonacci(n - 1, memo) + fibonacci(n - 2, memo)
return memo[n]
}
console.log(fibonacci(10)) // Output: 55
Pros:
- Efficient: time complexity of O(n)
- Elegant code
Cons:
- Not as straightforward as the iterative approach
- Slightly more complex implementation
Hi there! Want to support my work?
The Fibonacci series is a popular programming problem that showcases the versatility of JavaScript and the power of modern ES6+ features. Each approach has its benefits and drawbacks, but the memoization technique offers an elegant and efficient solution for solving the Fibonacci series. By understanding and implementing these different methods, you can deepen your knowledge of JavaScript and improve your problem-solving skills in coding challenges.