Mastering Merge Intervals - A Comprehensive Guide to Combining Overlapping Intervals

- Published on
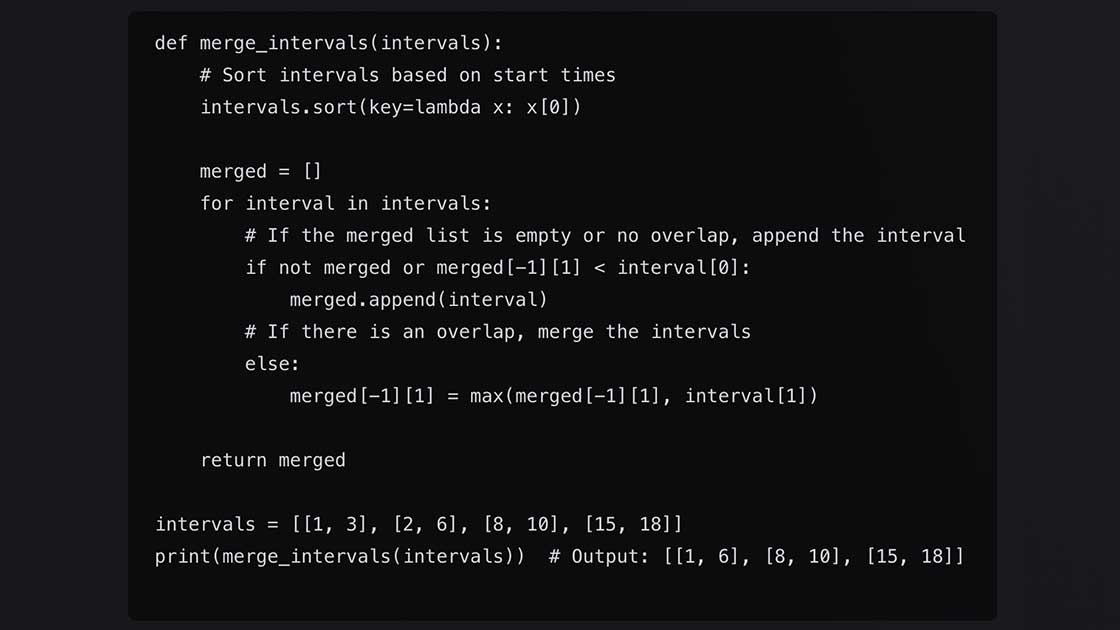
In computer science and programming, merge intervals is a common problem that deals with combining overlapping intervals in a given set. This problem frequently appears in coding interviews and challenges, making it essential for developers to understand the concept and various approaches to solving it. In this article, we will explore the merge intervals problem, discuss its applications, and walk through a step-by-step solution using Python.
Problem Statement
Given a collection of intervals, merge any overlapping intervals. For example:
Input: intervals = [[1, 3], [2, 6], [8, 10], [15, 18]] Output: [[1, 6], [8, 10], [15, 18]]
Explanation: Since intervals [1, 3] and [2, 6] overlap, they are merged into [1, 6].
Approach and Algorithm
Sort the intervals based on their start times. Initialize an empty list (merged) to store the merged intervals. Iterate through the sorted intervals. If the merged list is empty or the current interval does not overlap with the previous one, append the current interval to the merged list. If the current interval overlaps with the previous one, merge the intervals by updating the end time of the previous interval.
Python Solution
def merge_intervals(intervals):
# Sort intervals based on start times
intervals.sort(key=lambda x: x[0])
merged = []
for interval in intervals:
# If the merged list is empty or no overlap, append the interval
if not merged or merged[-1][1] < interval[0]:
merged.append(interval)
# If there is an overlap, merge the intervals
else:
merged[-1][1] = max(merged[-1][1], interval[1])
return merged
intervals = [[1, 3], [2, 6], [8, 10], [15, 18]]
print(merge_intervals(intervals)) # Output: [[1, 6], [8, 10], [15, 18]]
Applications
The merge intervals problem has various real-world applications, including:
Calendar and scheduling systems: Merge intervals can be used to find free time slots or determine overlapping appointments and events. Resource allocation: In systems where resources have time-based availability, merge intervals can help identify gaps or overlaps in the allocation. Genome sequencing and bioinformatics: Merge intervals can be used to identify overlapping genetic sequences or regions of interest in DNA data.
Hi there! Want to support my work?
The merge intervals problem is a fundamental coding challenge that developers should be familiar with to tackle similar problems in coding interviews and real-world applications. By understanding the problem, following the algorithm, and practicing the implementation, you can improve your problem-solving skills and deepen your knowledge of Python or other programming languages. Keep practicing, and remember to apply the same approach to other interval-based problems you encounter in your coding journey!